Exercise: Write, compile and run a Java program
3. Exercise: Write, compile and run a Java program
3.1. Write source code
The following Java program is developed under Linux using a text editor and the command line. The process on other operating system should be similar, but is not covered in this description.
Select or create a new directory which will be used for your Java development. In this description the path\home\vogella\javastarter
is used. On Microsoft Windows you might want to use c:\temp\javastarter
. This path is called javadir in the following description.
Open a text editor which supports plain text, e.g., gedit under Linux or Notepadunder Windows and write the following source code.
// a small Java program
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello World");
}
}
Do not use a rich editor like Microsoft Word or LibreOffice for writing Java code. If in doubt, google for "Plain text editor for [your_OS]". |
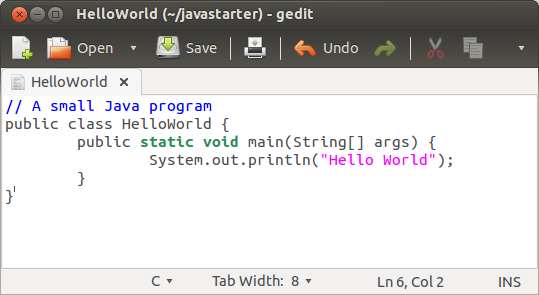
Save the source code in your javadirdirectory with the HelloWorld.java
filename. The name of a Java source file must always equal the class name (within the source code) and end with the .java
extension. In this example the filename must beHelloWorld.java
, because the class is called HelloWorld
.
3.2. Compile and run your Java program
Open a shell for command line access.
If you don’t know how to do this, google for "How to open a shell under [your_OS]". |
Switch to the javadir directory with the command cd javadir
, for example, in the above example via the cd \home\vogella\javastarter
command. Use the ls
command (dir
under Microsoft Windows) to verify that the source file is in the directory.
Compile your Java source file into a class file with the following command.
javac HelloWorld.java
Afterwards list again the content of the directory with the ls
or dir
command. The directory contains now a file HelloWorld.class
. If you see this file, you have successfully compiled your first Java source code into bytecode.
By default, the compiler puts each class file in the same directory as its source file. You can specify a separate destination directory with the -d compiler flag. |
You can now start your compiled Java program. Ensure that you are still in thejardir directory and enter the following command to start your Java program.
java HelloWorld
The system should write "Hello World" on the command line.
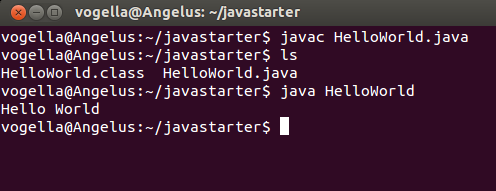
3.3. Using the classpath
You can use the classpath to run the program from another place in your directory.
Switch to the command line, e.g., undercmd
. Switch to any directory you want. Type:
java HelloWorld
If you are not in the directory in which the compiled class is stored, then the system will show an error message:Exception in thread "main" java.lang.NoClassDefFoundError: test/TestClass
To use the class, type the following command. Replace "mydirectory" with the directory which contains the test directory. You should again see the "HelloWorld" output.
java -classpath "mydirectory" HelloWorld
Comments
Post a Comment